How to remove “Chrome is being controlled by automated test software” ?
Let’s say you create a bot that instrument a Chrome browser with Puppeteer using the code below:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({
executablePath: '/Applications/Google Chrome.app/Contents/MacOS/Google Chrome',
headless: false
});
const page = await browser.newPage();
await page.goto('https://deviceandbrowserinfo.com/http_headers');
await browser.close()
})();
You may notice that Chrome adds the following warning,
Chrome is being controlled by automated test software
, under the URL bar (cf screenshots
below).
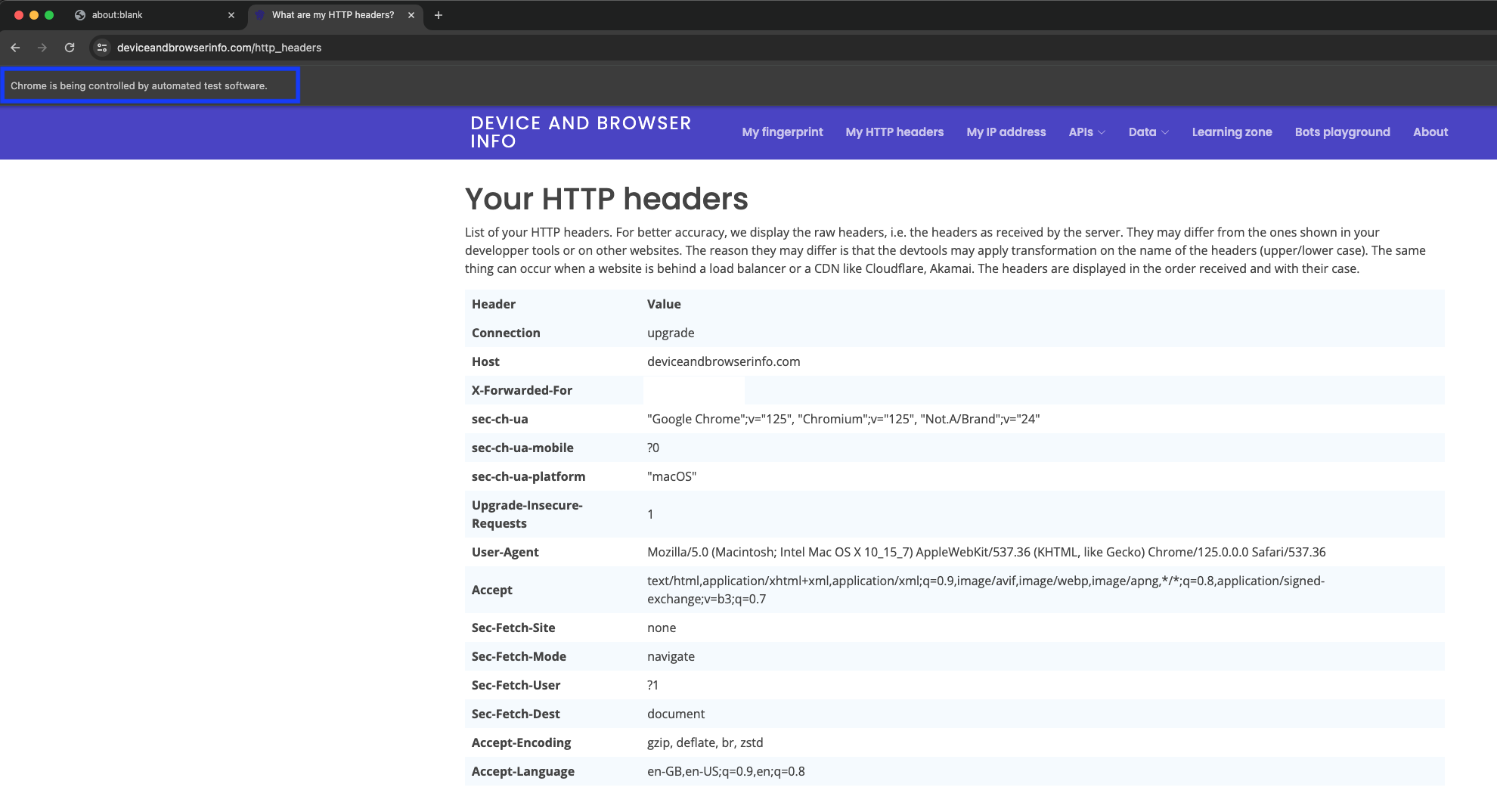
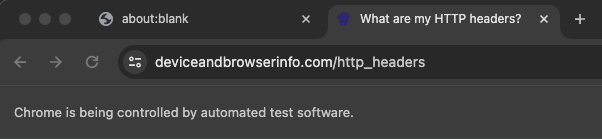
To remove the
Chrome is being controlled by automated test software
warning, you need to pass the
ignoreDefaultArgs: ["--enable-automation"]
parameter when creating the puppeteer
browser instance:
const browser = await puppeteer.launch({
executablePath: '/Applications/Google Chrome.app/Contents/MacOS/Google Chrome',
headless: false,
ignoreDefaultArgs: ["--enable-automation"]
});
This parameter removes the
Chrome is being controlled by automated test software
warning (cf screenshot below). However,
you should note that it doesn’t make your bot undetectable.
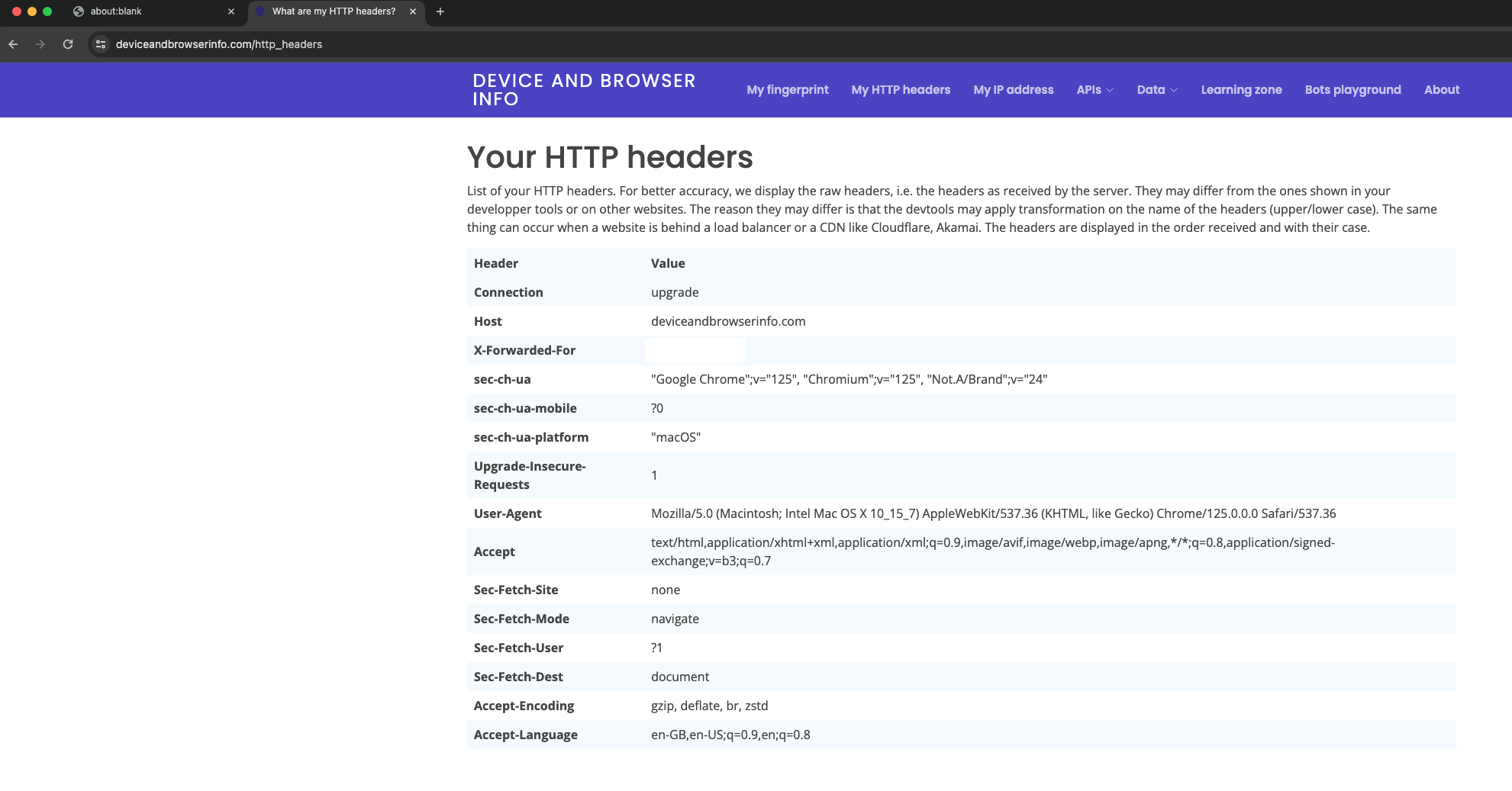